- Published on
Creating Automated API Tests with Postman: Part 1
- Authors
- Name
- Scottie Crump
- @linkedin/scottiecrump/
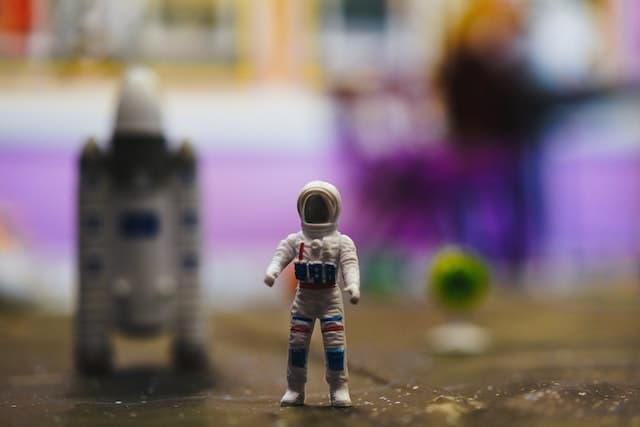
Photo by Phil Hearing on Unsplash
Overview
Postman is a popular tool to test APIs. Many people use Postman to test various endpoints quickly, but we can also create fully automated test suites. The application we will test in this blog series is a Client Manager built with Next.js. We will use a containerized version scottiecrump/client-address-next to get the application up and running quickly on our machine. Next, we will set up the application and review the API to test in the following sections.
Running the API Application with Docker
We will use Docker, a popular tool that bundles your application with all the libraries and services it depends on into a container package. The container is like a shipping container because you can run it on any machine long as it uses Docker. Docker handles problems such as, "It works on my machine, why not yours?" or "I want to run your application, but not have to install all the dependencies on my machine."
Use the "get started" instructions to install Docker on your machine. In addition, if you are using VSCode, consider installing the Docker extension. Once Docker is installed, create a new npm project and add a docker-compose.yml
file in the root of the project with the following code:
version: '3.8'
services:
api:
image: scottiecrump/client-address-next
environment:
- MONGO_URI="mongodb://mongo:27017/addressbook"
depends_on:
- mongo
ports:
- 3000:3000
restart: always
mongo:
image: mongo
ports:
- 27017:27017
volumes:
- mongo-data:/data/db
- mongo-config:/data/configdb
restart: always
volumes:
mongo-data:
driver: local
mongo-config:
driver: local
In the previous code, first, we specified the version of Docker Compose version: '3.8'
to use. Next, we use services
to identify the names of the containers we want to be built (i.e., api
and mongo
) and instructions.
For the api
service:
api:
image: scottiecrump/client-address-next
environment:
- MONGO_URI="mongodb://mongo:27017/addressbook"
depends_on:
- mongo
ports:
- 3000:3000
restart: always
we use the scottiecrump/client-address-next image, the API application itself. Next, we set the value for MONGO_URI
to connect to the Mongo database for environment
. Note the use of mongo
in the URL. Using "mongo" connects api
to the MongoDB service by its name. Next, we specify ports
to 3000:3000
. The port
specifies the port you want to expose in colon-separated values where the first port is your local machine and the second is the port specified inside the container. Then, we set restart
to always
to always restart the container if it crashes for some reason.
For the mongo
service:
mongo:
image: mongo
ports:
- 27017:27017
volumes:
- mongo-data:/data/db
- mongo-config:/data/configdb
restart: always
first, we set image
to mongo
to use the MongoDB Docker image. Next, we make the service available to the api
service by setting ports
to 27107:27107
. Next, we use volumes
to persist data saved to the database. For example, if we don't use volumes
, any data stored in the database will be lost if we stop and restart the container. However, when we use volumes
, we can stop and restart the container, and that data persists. The volume
paths are prepended with the labels mongo-data
and mongo-config
, allowing them to be easily identified among other volumes that may be running on our machine. Next, we set restart
to always
to handle crashes. Finally, we specify all the named volumes
sharable with other services using the default local
driver
.
Start the containers using the following command:
docker-compose up -d
Stop the containers using the following command:
docker-compose down
API Endpoint Overview
The API has five endpoints:
- POST
/api/add-client
used to create new clients - GET
/api/clients
to retrieve all current clients - GET
/api/get-client?id
to retrieve a single client - POST
/api/update-client
to update a single client - DELETE
/api/delete-client
to delete a single client
Detailed API documentation can be found here, including example requests with responses.
Part 1 Review
In review, we learned how to start the API to test using Docker and looked at the documentation for the API. In the next section, we will start testing the API using Postman.