- Published on
ES6 TypeScript: Part 2
- Authors
- Name
- Scottie Crump
- @linkedin/scottiecrump/
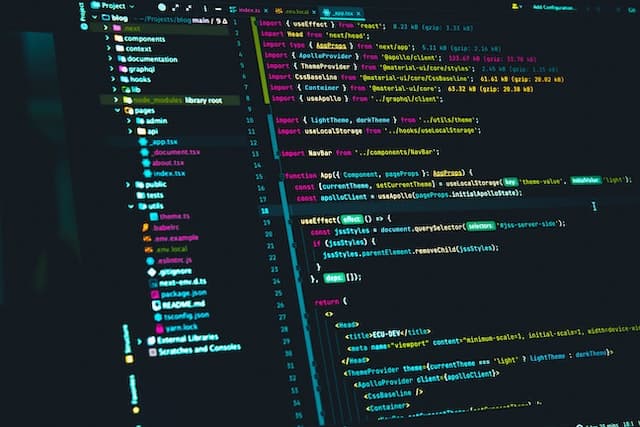
Photo by Juanjo Jaramillo on Unsplash
Part 2
In part 2 of the series, we will look at the every
, some
, reduce
methods and template literals.
every Method
The every
method returns true
if all array values match the callback function criteria. Otherwise, the returned result is false
.
Example 1
const students = [
{name: 'Brad',age: 19 major: 'Computer Science'}
{name: 'Sally',age: 20 major: 'Art'}
{name: 'Michelle',age: 22 major: 'Math'}
{name: 'Joe',age: 21 major: 'Computer Science'}
{name: 'Karen',age: 19 major: 'Computer Science'}
]
const isEveryStudentOver20 = students.every(function(student: {
name: string
age: number
}): boolean {
return student.age > 20
})
console.log(isEveryStudentOver20) // false
The following video illustrates the examples:
Part 3
some Method
The some
method returns true
if at least one array value matches the callback function criteria. Otherwise, the returned result is false
.
Example 1
const students = [
{name: 'Brad',age: 19 major: 'Computer Science'}
{name: 'Sally',age: 20 major: 'Art'}
{name: 'Michelle',age: 22 major: 'Math'}
{name: 'Joe',age: 21 major: 'Computer Science'}
{name: 'Karen',age: 19 major: 'Computer Science'}
]
const areSomeOver20 = students.every(function(student: {
name: string
age: number
}): boolean {
return student.age > 20
})
console.log(areSomeOver20) // true
The following video illustrates the examples:
reduce Method
The reduce
method returns a single value from an array that is reduced based on the callback function criteria.
Example 1
const studentScores = [80, 90, 85, 75, 100]
const scoresSum = studentScores.reduce(function (total: number, currVal: number): number {
return total + currVal
}, 0)
console.log(scoresSum) // 430
Example 2
const studentScores = [80, 90, 85, 75, 100]
function getAvg(total: number, currVal: number): number {
return total + currVal
}
const averageScore = studentScores.reduce(getAvg) / studentScores.length
console.log(scoresSum) // 86
The following video illustrates the examples:
Template Literals
Template literals (also known as template strings) are a cleaner way of creating string outputs that include embedded variable values.
Example 1
function printCurrentDate(): string {
const month = new Date().getMonth()
const date = new Date().getDate()
const year = new Date().getFullYear()
return `Current date is ${month}/${date}/${year}`
}
console.log(printCurrentDate)
The following video illustrates the examples: