- Published on
ES6 TypeScript: Part 1
- Authors
- Name
- Scottie Crump
- @linkedin/scottiecrump/
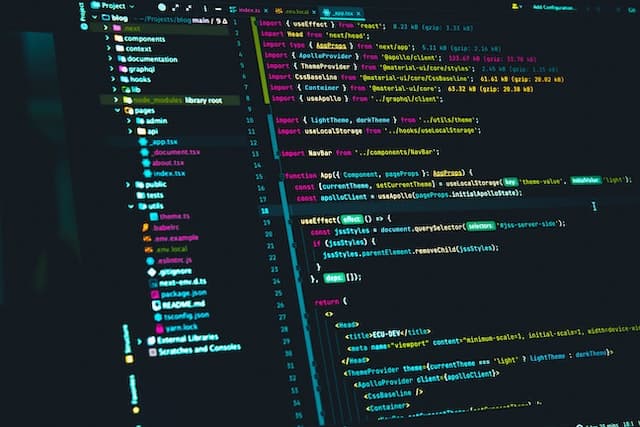
Photo by Juanjo Jaramillo on Unsplash
Overview
I created a four part video series illustrating various ways to write JavaScript using ES6 syntax. Associated code snippets and videos are linked with each blog.
Part 1
In part 1 of the series, we will look at the forEach
, map
, filter
, and find
methods.
forEach Method
The forEach method is a replacement for the classic for loop
. Note that the forEach
method does not return a value. So, for example, if you want to iterate over an array to edit the values, you need to store the new values in a separate variable.
Example 1
const numbers = [1, 2, 3, 4, 5]
let sum = 0
numbers.forEach(function (num: number): void {
sum += num
})
console.log(sum) // 15
Example 2
const numbers = [1, 2, 3, 4, 5]
let sum = 0
function sumNums(num: number): void {
sum += num
}
numbers.forEach(sumNums)
console.log(sum) // 15
The following video illustrates the examples:
map Method
The map
method allows you to iterate over an existing array and return a new array based on modifying current values via the call-back function.
Example 1
const digits = [1, 2, 3]
const doubled = digits.map(function (digit: number): number {
return digit * 2
})
console.log(doubled) // [2,4,6]
Example 2
const cars = [
{ model: 'Buick', price: 'cheap' },
{ model: 'Camaro', price: 'expensive' },
]
const prices = cars.map(function (car: { model: string; price: string }): string {
return cars.price
})
console.log(prices) // ['cheap', 'expensive']
The following video illustrates the examples:
filter Method
The filter
method allows you to iterate over an existing array and return a new array with filtered results matching the pattern in the callback function.
Example 1
const students = [
{name: 'Brad', major: 'Computer Science'}
{name: 'Sally', major: 'Art'}
{name: 'Michelle', major: 'Math'}
{name: 'Joe', major: 'Computer Science'}
{name: 'Karen', major: 'Computer Science'}
]
const CSMajors = students.filter(function(student: {
name: string
major: string
}): boolean {
return student.major === 'Computer Science'
})
console.log(CSMajors)
/*
[
{name: 'Brad', major:'Computer Science'}
{name: 'Joe', major: 'Computer Science'}
{name: 'Karen', major: 'Computer Science'}
]
*/
The following video illustrates the example:
find Method
The find
method allows you to iterate over an array and return the first result found that matches the pattern in the callback function.
Example 1
const students = [
{name: 'Brad',age: 19 major: 'Computer Science'}
{name: 'Sally',age: 20 major: 'Art'}
{name: 'Michelle',age: 22 major: 'Math'}
{name: 'Joe',age: 21 major: 'Computer Science'}
{name: 'Karen',age: 19 major: 'Computer Science'}
]
const studentOver20 = students.find(function(student: {
name: string
age: number
}): string | undefined {
if(student.age > 20) {
return student.name
}
})
console.log(studentOver20) // {name: 'Michelle',age: 22 major: 'Math'}
The following video illustrates the example: