- Published on
Test a Feedback Form: Part 5
3 min read | 589 words
- Authors
- Name
- Scottie Crump
- @linkedin/scottiecrump/
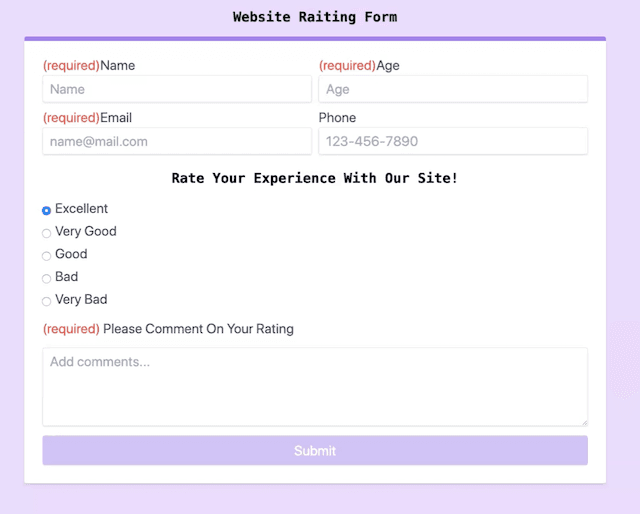
Part 5
In part 5 of the series, we will use Postman to test API routes for the feedback form.
Postman API Tests
POST /api/add-feedback Route
Request Body
{
"name": "Tom Jones",
"age": "33",
"email": "t344j@mail.com",
"rating": "excellent",
"comment": "Sample comment"
}
New Entry Response Body
status 200
{
"message": "feedback for Tom Jones successfully added!"
}
Duplicate Entry Response Body
status 409
{
"message": "feedback for t344j@mail.com already received"
}
POST /api/add-feedback Route Missing Data Response
Request Body
{
"name": "Tom Jones",
"age": "33",
"rating": "excellent",
"comment": "Sample comment"
}
Response Body
status 422
{
"message": "Missing 1 or more required fields and/or valid phone number"
}
The following video illustrates part 5:
Summary
In summary, we wrote several tests to verify the functionality of the feedback form. We wrote component tests using React Testing Library and API tests using Postman. As a bonus, a Cypress test not illustrated in video form is included below to verify expected behavior via the UI:
Test Data Utility File
import faker from 'faker'
const cyRatings = ['excellent', 'veryGood', 'good', 'bad', 'veryBad']
const ratings = ['excellent', 'veryGood', 'good', 'bad', 'veryBad']
export default {
name: faker.name.findName(),
age: faker.random.number(99),
email: faker.internet.email(),
phone: faker.phone.phoneNumberFormat(),
cyRating: cyRatings[Math.floor(Math.random() * ratings.length)],
rating: ratings[Math.floor(Math.random() * ratings.length)],
comment: faker.random.words(10),
}
Cypress Feedback Flow Test
import testData from '../../utils/testData'
describe('Add Feedback Form', () => {
it('When required fields submitted then feedback is added', () => {
cy.visit('/')
cy.findByLabelText(/name/i).type(testData.name).clear()
cy.findByText(/Please enter a name/i).should('be.visible')
cy.findByLabelText(/name/i).type(testData.name)
cy.findByLabelText(/age/i).type(testData.age).clear()
cy.findByText(/Please enter an age/i).should('be.visible')
cy.findByLabelText(/age/i).type(testData.age)
cy.findByLabelText(/email/i).type(testData.email).clear()
cy.findByText(/Please enter an email/i).should('be.visible')
cy.findByLabelText(/email/i).type(testData.email)
cy.findByLabelText(/phone/i).type(testData.phone)
cy.findByTestId(testData.cyRating).click()
cy.findByLabelText(/comment/i)
.type(testData.comment)
.clear()
cy.findByText(/Please enter a comment/i).should('be.visible')
cy.findByLabelText(/comment/i).type(testData.comment)
cy.findByText(/submit/i).click()
cy.url().should('contain', 'thanks')
cy.findByText(/go home/i).click()
cy.findByText(/Website Raiting Form/i).should('be.visible')
})
})